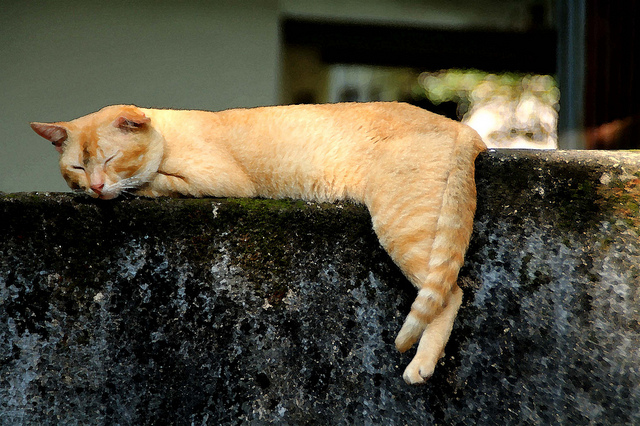
Influxdb is open source database which is predominantly used for time series data collection . I have been using influxdb on my raspberry pi to store data from IoT sensors at home. So far I was using python influxdb module to pump in data. Recently I wanted to find some clientless solution to manage data in influxdb. And finally I have managed to publish and retrieve data using simple REST calls to influxdb.
Let us take deep dive to implement this solution. It is always a good idea to protect any API access with authentication, so let us first create an admin level user in influxdb using influxdb command line interface.
CREATE USER infxadmin WITH PASSWORD 'infxpass' WITH ALL PRIVILEGES
Now to expose influxdb on REST, enable influxdb HTTP interface with authentication by adding following lines in influxdb.conf file. This will enable HTTP interactions with influxdb on port 8086.
[http]
enabled = true
bind-address = "0.0.0.0:8086"
auth-enabled = true
log-enabled = true
write-tracing = false
pprof-enabled = false
access-log-path = "/var/log/influxdb/access.log"
On one terminal keep watching activities in /var/log/influxdb/access.log
tail -f /var/log/influxdb/access.log
Restart influxdb daemon
systemctl restart influxdb
Connect to influxdb CLI using login/password
influx -username infxadmin -password ''
password:
Connected to http://localhost:8086 version 1.7.4
InfluxDB shell version: 1.7.4
Verify if you are able to perform database operations as infxadmin user. for eg, simply try to list databases.
> show databases
name: databases
name
----
_internal
Now, Create a database and another database user for this new database
> create database testdb
> create user testuser with password 'testpass' with all privileges
> grant all on testdb to testuser
Fire REST call to list databases.
curl -G http://localhost:8086/query -u infxadmin:infxpass --data-urlencode "q=SHOW DATABASES"
{"results":[{"statement_id":0,"series":[{"name":"databases","columns":["name"],"values":[["_internal"],["testdb"]]}]}]}
Next, we will insert voltage and current readings of electrical point in “energy_tracker” measurement within “testdb” database. You can change measurement name and fields according to your project.
curl -i -XPOST 'http://localhost:8086/write?db=testdb' -u testuser:testpass --data-binary 'energy_tracker,elec_point=kitchen_light voltage=200,current=0.3'
HTTP/1.1 204 No Content
Content-Type: application/json
Request-Id: 2bb4be13-4163-11e9-801b-0050568faa8d
X-Influxdb-Build: OSS
X-Influxdb-Version: 1.7.4
X-Request-Id: 2bb4be13-4163-11e9-801b-0050568faa8d
Date: Fri, 08 Mar 2019 05:29:40 GMT
Looks good so far. Let us verify if measurement “energy_tracker” is created and record is inserted in measurement
> show measurements
name: measurements
name
----
energy_tracker
> select * from energy_tracker
name: energy_tracker
time current elec_point voltage
---- ------- ---------- -------
1552022980234444207 0.3 kitchen_light 200
You can use this approach to push/pull data from/to influx using any programming language supporting REST.