I have basic CCTV setup at home with two bullet cameras on 4 channel hikvision DVR. Hikvision DVR does not offers face detection, vehicle detection or any other image processing functions with basic cameras. I thought it will be worth to get CCTV feeds in python and then do try out face detection etc on the streams.
You can do this with any CCTV/DVR setup. First you need to figure out the RTSP url of your DVR. In case of hikvision stream URLs are like:
rtsp://username:password@<dvr_ip_address>:554/Streaming/Channels/101 - Ch 1 HD stream
rtsp://username:password@<dvr_ip_address:554/Streaming/Channels/102 - Ch 1 basic stream
rtsp://username:password@<dvr_ip_address:554/Streaming/Channels/301 - Ch 3 HD stream
rtsp://username:password@<dvr_ip_address:554/Streaming/Channels/302 - Ch 3 basic stream
You should first test RTSP stream in a network stream video player, such as VLC player. Once you see CCTV stream output on player then you are ready to get it in python.
Using opencv module you can get the stream and do a lot of stuff on top of the stream. Let us just try to get stream working now. You have to insall opencv module as following
sudo pip3 install opencv-python
Now, using opencv module you can read stream from RTSP urls and display frames using following code.
#!/usr/bin/python3
#Import opencv module
import cv2
# Get streams from RTSP urls of DVR
door = cv2.VideoCapture("rtsp://username:password@<dvr_ip_address>:554/Streaming/Channels/101")
parking = cv2.VideoCapture("rtsp://username:password@<dvr_ip_address>:554/Streaming/Channels/302")
# Go in infinite loop to show frames from stream
while (1):
ret, frame = door.read()
cv2.imshow('Main Door', frame)
ret, frame = parking.read()
cv2.imshow('Parking', frame)
key = cv2.waitKey(33)
if key==27: # press ESC to quit
break
elif key==-1: # normally -1 returned,so don't print it
continue
else:
print(key)
Upon executing above program you will see streams like this
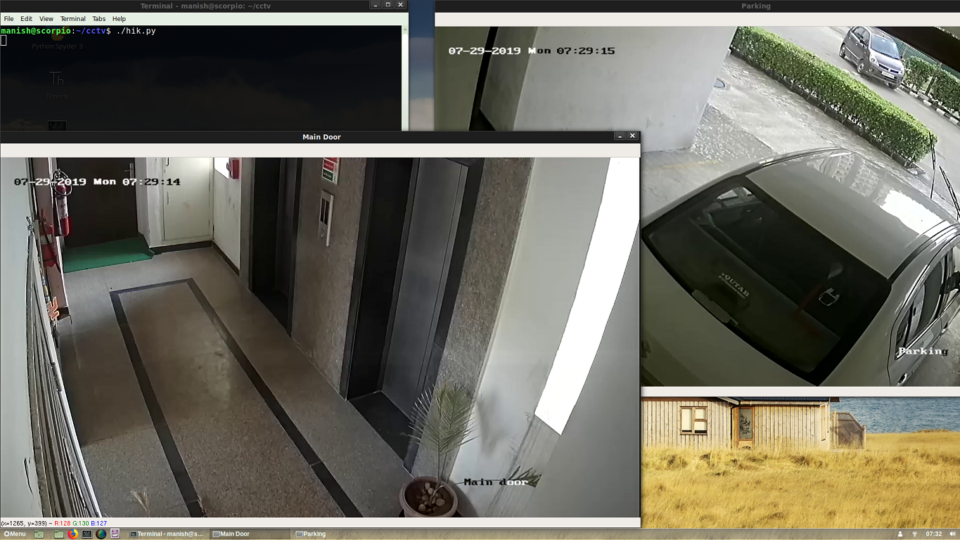